In this Article we will be dropping the Csc 231 Past Questions and a the Answers, Access then Below. if you didn’t see the complete answers use the WhatsApp button to contact our admins.
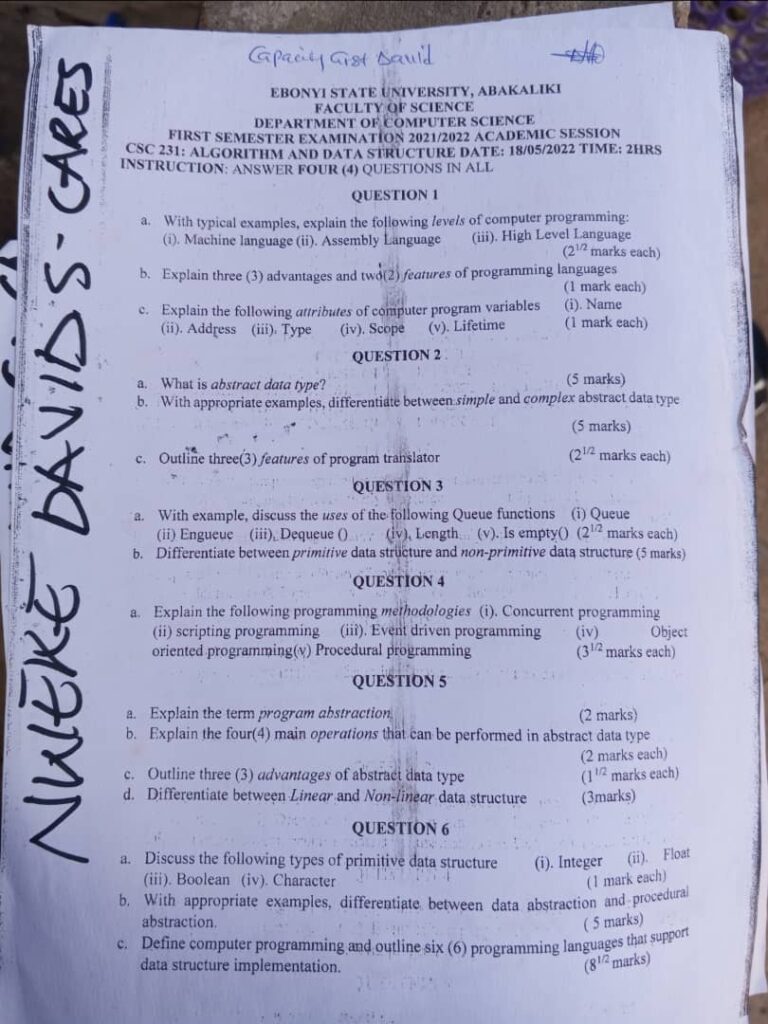
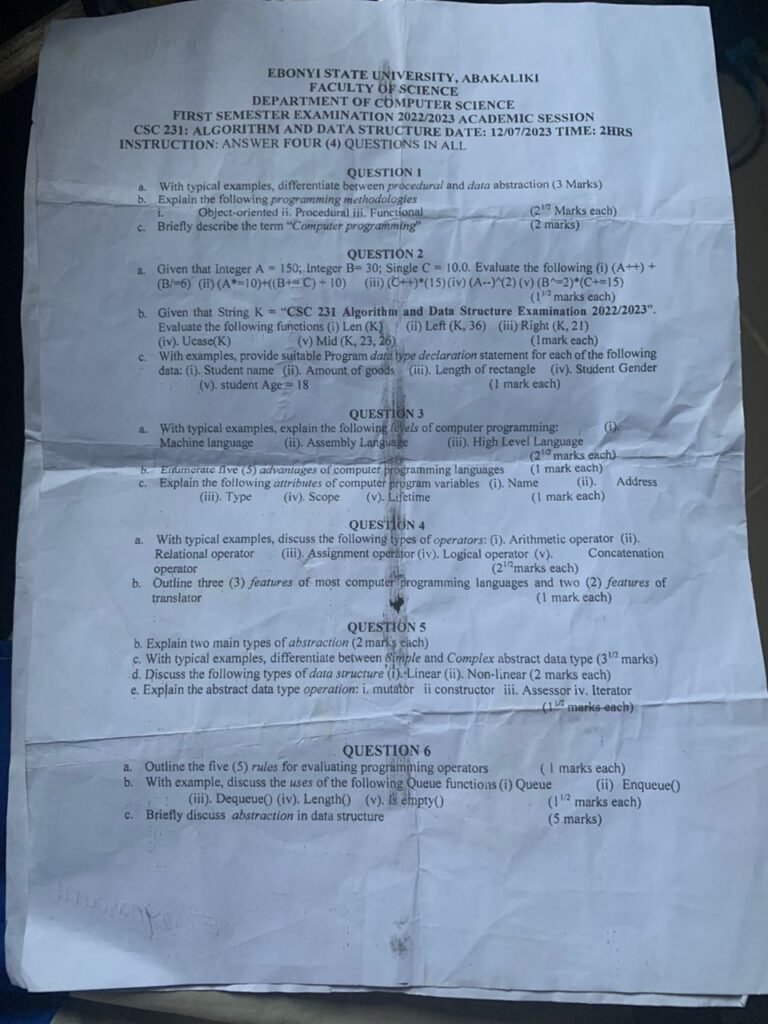
SOLUTIONs
1
(i) Machine Language: This is the lowest level of programming language. It consists of binary code, which is directly executed by the computer’s hardware. An example of machine language is 0101010101010101.
(ii) Assembly Language: This is a low-level programming language that is more readable than machine language. It uses symbols to represent the instructions that are executed by the computer’s hardware. An example of assembly language is ADD AX, BX, which adds the contents of the AX and BX registers.
(iii) High-Level Language: This is a programming language that is closer to human language and is easier to read and write than low-level languages. High-level languages like Python, Java, and C++ are designed to be more user-friendly and abstracted away from the hardware. An example of high-level language is “print(‘Hello, World!’)” in Python, which prints the text “Hello, World!” to the console.
B
Sure, here are brief explanations of the advantages and features of programming languages:
Advantages:
- Efficiency: Programming languages allow developers to create efficient and optimized code that can perform complex tasks quickly and accurately.
- Portability: Programs written in high-level languages can be run on different hardware and operating systems without modification, making them highly portable.
- Maintainability: Programming languages allow developers to easily modify and update their code, making it easier to maintain and fix errors.
Features:
- Syntax: Programming languages have a specific syntax that must be followed in order for the code to be executed properly. This includes the use of keywords, operators, and punctuation.
- Data Types: Programming languages have different data types that can be used to store and manipulate data. These include integers, floats, strings, and booleans, among others.
C
Sure, here are brief explanations of the attributes of computer program variables:
(i) Name: This is the identifier used to represent a variable in a program. It can be any combination of letters, numbers, and special characters, but must follow certain rules depending on the programming language.
(ii) Address: This is the memory location where the value of a variable is stored. Every variable has a unique address in memory that can be accessed by the program.
(iii) Type: This refers to the data type of a variable, such as integer, float, or string. The type determines the size and format of the data that can be stored in the variable.
(iv) Scope: This refers to the part of the program where a variable can be accessed. Variables can have global or local scope, depending on where they are declared in the program.
(v) Lifetime: This refers to the duration of time that a variable exists in memory. Variables can have static or dynamic lifetime, depending on how they are declared and used in the program.
2
a) An abstract data type is a data type that is defined by its behavior rather than its implementation. It is a high-level description of a data type that specifies the operations that can be performed on it and the constraints that apply to those operations. Abstract data types are used to encapsulate data and provide a well-defined interface for working with that data.
b) Simple abstract data types are those that are built into a programming language and are not composed of other data types. Examples of simple abstract data types include integers, floats, characters, and booleans.
Complex abstract data types, on the other hand, are composed of other data types and are defined by their behavior rather than their implementation. Examples of complex abstract data types include arrays, lists, stacks, and queues. These data types provide a way to organize and manipulate large amounts of data in a structured and efficient manner. They are typically implemented using simple abstract data types and provide a higher-level interface for working with that data.
C
here are three features of program translators:
- Translation: The primary feature of program translators is their ability to translate code from one language to another. This includes compiling source code into object code, interpreting code on-the-fly, or translating code from one programming language to another.
- Optimization: Program translators often include optimization features that can improve the efficiency and performance of the code being translated. This can include techniques such as code simplification, loop unrolling, and register allocation.
- Error Checking: Program translators typically include error checking features that can detect and report errors in the code being translated. This can include syntax checking, type checking, and semantic checking to ensure that the code is valid and will execute correctly.
3
a. Here are the uses of the following Queue functions:
(i) Queue: This function creates a new Queue data structure. For example, you can create a new Queue to store a list of tasks that need to be completed.
(ii) Enqueue: This function adds an item to the end of the Queue. For instance, you can use this function to add new tasks to the list of tasks that need to be completed.
(iii) Dequeue: This function removes and returns the item at the front of the Queue. For example, you can use this function to remove the first task from the list of tasks that need to be completed.
(iv) Length: This function returns the number of items in the Queue. For example, you can use this function to determine how many tasks are left to be completed.
(v) Is empty(): This function returns True if the Queue is empty and False otherwise. For example, you can use this function to check if there are any tasks left to be completed.
b. Here is the difference between primitive data structures and non-primitive data structures:
Primitive data structures are basic data types that are built into a programming language. They include integers, floats, characters, and booleans. These data types are simple and can be represented using a fixed number of bits. They are typically used to store small amounts of data and perform basic operations such as arithmetic and logical operations.
Non-primitive data structures, on the other hand, are more complex data types that are composed of other data types. They include arrays, lists, stacks, and queues. These data types can store large amounts of data and provide a way to organize and manipulate that data in a structured and efficient manner. They are typically used to represent more complex data structures such as databases, trees, and graphs.
4
Here’s an explanation of the following programming methodologies:
- Concurrent programming: Concurrent programming is a programming methodology that deals with the execution of multiple tasks at the same time. It is commonly used in systems that require high performance and responsiveness, such as operating systems and real-time applications. Concurrent programming can be achieved through the use of threads, processes, or coroutines.
- Scripting programming: Scripting programming is a programming methodology that involves writing scripts to automate tasks or to add functionality to an existing program. Scripts are typically interpreted rather than compiled, and are often used to automate repetitive tasks, such as file processing, system administration, and web development.
- Event-driven programming: Event-driven programming is a programming methodology that responds to user actions or system events. It is commonly used in graphical user interfaces (GUIs) and web applications, where user input triggers events that cause the program to perform certain actions. Event-driven programming typically involves the use of callbacks and event handlers.
- Object-oriented programming: Object-oriented programming (OOP) is a programming methodology that focuses on the creation of objects, which are instances of classes that encapsulate data and behavior. OOP is based on the principles of abstraction, encapsulation, inheritance, and polymorphism, and is commonly used in a variety of applications, such as games, web applications, and enterprise systems.
- Procedural programming: Procedural programming is a programming methodology that focuses on the creation of procedures, which are sequences of instructions that perform a specific task. Procedural programming is typically used in applications that require a step-by-step approach to problem-solving, such as scientific computing and numerical analysis.
5
Here’s an explanation of the following programming concepts:
a) Program Abstraction: Program abstraction is a programming technique that involves hiding the implementation details of a program and exposing only the necessary details to the user. It allows programmers to create complex systems by breaking them down into smaller, more manageable pieces. Abstraction is achieved through the use of interfaces, classes, and modules, which provide a high-level view of the program’s functionality without exposing the underlying details.
b) Abstract Data Type (ADT): An abstract data type is a data type that is defined by its behavior, rather than its implementation. It provides a high-level view of the data and the operations that can be performed on it, without exposing the underlying details. The four main operations that can be performed on an ADT are:
- Creation: This operation involves creating a new instance of the ADT. The creation operation typically involves allocating memory and initializing the data.
- Insertion: This operation involves adding a new element to the ADT. The insertion operation typically involves checking for duplicates and maintaining the order of the data.
- Deletion: This operation involves removing an element from the ADT. The deletion operation typically involves searching for the element and updating the data structure.
- Traversal: This operation involves accessing all the elements in the ADT. The traversal operation typically involves iterating through the data structure and performing some operation on each element.
c) Here are three advantages of abstract data type:
- Reusability: Abstract data types can be reused in multiple programs, which saves time and effort. Once an ADT is defined, it can be used in different programs without having to redefine it.
- Encapsulation: Abstract data types encapsulate the data and the operations that can be performed on it. This means that the implementation details are hidden from the user, which makes the program easier to use and maintain.
- Abstraction: Abstract data types provide a high-level view of the data and the operations that can be performed on it. This means that the user can focus on the functionality of the program, rather than the implementation details.
d) Linear data structures and non-linear data structures are two types of data structures in computer science. Here’s the difference between them:
- Linear data structures: Linear data structures are data structures in which the elements are arranged in a linear sequence, such as a linked list or an array. The elements in a linear data structure are accessed in a sequential order, which means that each element has a unique predecessor and successor.
- Non-linear data structures: Non-linear data structures are data structures in which the elements are not arranged in a linear sequence, such as a tree or a graph. The elements in a non-linear data structure are accessed in a non-sequential order, which means that each element may have multiple predecessors and successors. Non-linear data structures are more complex than linear data structures, but they can represent more complex relationships between the elements.
6a
Here’s a brief explanation of each of the primitive data types:
(i) Integer: An integer is a whole number that can be positive, negative, or zero. In programming, integers are usually represented using a fixed number of bits, which determines the range of values that can be stored. For example, an 8-bit integer can store values from -128 to 127, while a 32-bit integer can store values from -2,147,483,648 to 2,147,483,647.
(ii) Float: A float is a data type that is used to represent decimal numbers. Floats are usually represented using a fixed number of bits, which determines the precision and range of values that can be stored. For example, a 32-bit float can store values with up to 7 decimal digits of precision, while a 64-bit float can store values with up to 15 decimal digits of precision.
(iii) Boolean: A boolean is a data type that can have one of two values: true or false. Booleans are often used in programming to represent logical values, such as whether a condition is true or false.
(iv) Character: A character is a data type that is used to represent a single letter, digit, or symbol. Characters are usually represented using a fixed number of bits, which determines the range of values that can be stored. For example, an 8-bit character can store 256 different values, which can represent the letters, digits, and symbols used in many different languages.
B
Data Abstraction: Data abstraction is a technique that is used to simplify complex data structures by hiding their implementation details and exposing only the relevant information to the user. This is typically done by defining a set of operations or functions that can be used to manipulate the data, while keeping the internal representation of the data hidden from the user. For example, consider a stack data structure. The user only needs to know that they can push and pop elements from the stack, without knowing how the stack is actually implemented.
Procedural Abstraction: Procedural abstraction is a technique that is used to simplify complex procedures or algorithms by hiding their implementation details and exposing only the relevant information to the user. This is typically done by defining a set of functions or procedures that can be used to execute the algorithm, while keeping the internal details of the algorithm hidden from the user. For example, consider a sorting algorithm. The user only needs to know that they can call a function to sort a list of elements, without knowing how the sorting algorithm is actually implemented.
c
Sure! Here’s a brief explanation of computer programming and six programming languages that support data structure implementation:
Computer Programming: Computer programming is the process of designing, writing, testing, and maintaining software programs. Programming is done using a programming language, which is a set of instructions that the computer can understand and execute. Programs can be written for a variety of purposes, such as automating tasks, solving complex problems, or creating interactive applications.
Six Programming Languages that Support Data Structure Implementation:
- C: C is a popular programming language that is widely used for system programming and embedded systems. It provides a rich set of data structures, such as arrays, linked lists, stacks, queues, and trees.
- C++: C++ is an object-oriented programming language that is an extension of C. It provides additional features, such as classes, templates, and operator overloading, which make it easier to implement complex data structures.
- Java: Java is a high-level programming language that is widely used for web development, mobile development, and enterprise applications. It provides a rich set of data structures, such as arrays, linked lists, stacks, queues, trees, and maps.
- Python: Python is a high-level programming language that is widely used for data analysis, machine learning, and scientific computing. It provides a rich set of data structures, such as lists, tuples, sets, dictionaries, and arrays.
- Ruby: Ruby is a high-level programming language that is widely used for web development and scripting. It provides a rich set of data structures, such as arrays, linked lists, stacks, queues, and trees.
- Swift: Swift is a programming language that is widely used for iOS and macOS development. It provides a rich set of data structures, such as arrays, linked lists, stacks, queues, and trees.